1. What is AngularJS? What are the advantages of AngularJS?
Answer:
AngularJS is an open-source JavaScript framework introduced by Google. It is a structural framework that helps to create dynamic Web apps. It helps to create extended HTML tags that can be used as normal HTML tags.
Advantages:
AngularJS is developed on MVC (Model-View-Controller) design pattern. You just have to split your application code into MVC components i.e. Model, View and the Controller. Views are pure html pages, and controllers written in JavaScript do the business processing.
AngularJS provides capability to create Single Page Application in a very clean and maintainable way
It supports dependency injection where components are given their dependencies instead of hard coding them within the component.
AngularJS code is unit testable.
All major browsers and smart phones including Android and iOS based phones/tablets support AngularJS applications.
2. What do you mean by Angular Expression?
Answer:
Angular expression is code snippets, usually placed in binding.
It can be written inside double braces, for eg. {{ angular_expression}} or can be written inside a directive, for eg. ng-bind="expression"
Example:
<!DOCTYPE html>
<html>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.4/angular.min.js"></script>
<body>
<div ng-app>
Addition:
{{ 7 + 5 }}
</div>
</body>
</html>
The above program is the simplest example of an Angular expression. The ng-app directive is blank, which means that there is no module to assign controllers, directives, services attached to the code. In the above program, we are adding a simple expression which performs the addition of two numbers.
3. What is injector in AngularJS?
Answer:
An injector is a service locator, used to retrieve object instance as defined by provider, instantiate types, invoke methods, and load modules.
Injectors inject your dependencies in your AngularJS application.
It creates an injector object that can be used for retrieving services as well as for dependency injection
Syntax:
angular.injector(modules, [strictDi]);
Example:
var someFunction = function($rootScope, $http)
{
return "called!";
};
app.run(function($rootScope, $injector)
{
$rootScope.annotations = $injector.annotate(someFunction);
$rootScope.message = $injector.invoke(someFunction);
});
The above code snippet demonstrates two capabilities of the $injector. First, you can use the annotate API to discover the dependency annotations for an injectable function (the above code would list "$rootScope" and "$http" as annotations).
And second, you can use the invoke API to execute an injectable function and have the $injector pass in the proper services.
4. What is meant by Internationalization in AngularJS?
Answer:
Internationalization is the process of developing products in such a way that they can be localized for languages and cultures easily.
To implement internationalization in AngularJS, it only requires to incorporate corresponding js according to locale of the country. By default it handles the locale of the browser.
AngularJS supports internationalization for date, number and currency filters.
5. Can we create nested controllers in AngularJS?
Answer:
Yes, we can create nested controllers in AngularJS. These controllers are defined in hierarchical manner while using in View.
Example:
<html>
<head>
<title>Angular JS Controller</title>
<script src = "https://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<h2>Sample Application</h2>
<div ng-app = "mainApp" ng-controller = "myController">
Enter first name: <input type = "text" ng-model = "emp.firstName"><br><br>
Enter last name: <input type = "text" ng-model = "emp.lastName"><br> <br>
You are entering: {{emp.fullName()}}
</div>
<script>
var mainApp = angular.module("mainApp", []);
mainApp.controller('myController', function($scope) {
$scope.emp = {
firstName: "Prajakta",
lastName: "Pandit",
fullName: function() {
var empObject;
empObject = $scope.emp;
return empObject.firstName + " " + empObject.lastName;
}
};
});
</script>
</body>
</html>
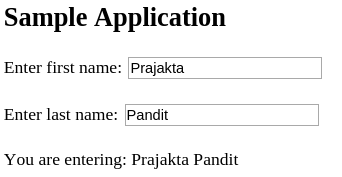