Exception Handling in Java
Exception
Exception is an event that arises during the execution of the program, and it terminates the program abnormally. It then displays system generated error message.
Exception Handling
Exception handling is the mechanism to handle the abnormal termination of the program.
For example :ClassNotFoundException, NumberFormatException, NullPointerException etc.
Need for Exception Handling
A program rarely executes without any errors for the first time. Users may run applications in unexpected ways. A program should be able to handle these abnormal situations.
- Exception handling allows executing the code without making any changes to the original code.
- It separates the error handling code from regular code.
Exception Hierarchy in Java
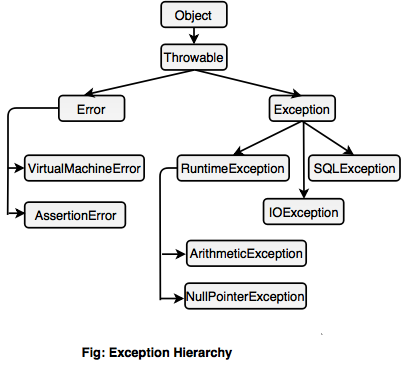
Difference between error and exception
Error | Exception |
---|
It cannot be caught. | It can be handled by try and catch block. |
Errors are by default unchecked type. | Exception can be either checked or unchecked type. |
It is defined in java.lang.Error package. | It is defined in java.lang.Exception package. |
Errors are generally caused by environment in which application is running. | Exceptions are generally caused by application itself. |
Example: StackOverflowError, OutOfMemoryError etc. | Example: SQLException, IOException, ClassCastException, ArrayOutOfBoundException |
Types of Exceptions
Java has two types of exceptions.
1. Checked exception
2. Unchecked exception
1. Checked Exceptions- Checked exceptions are also known as compiled time exception, because such exceptions occur at compile time.
- Java compiler checks if the program contains the checked exception handler or not at the time of compilation.
- All these exceptions are subclass of exception class.
- Developer has overall control to handle them.
For example: SQLException, IOException, ClassNotFoundException etc.
Example : Sample program for checked exception
import java.io.File;
import java.io.PrintWriter;
public class Checked_Demo
{
public static void main(String args[])
{
File file=new File("E://abc.txt");
PrintWriter pw = new PrintWriter("file");
}
}
Output:
error: FileNotFoundException
2. Unchecked Exceptions- Unchecked exceptions are also known as runtime exception.
- These include logic errors or improper use of API.
For example: ArrayIndexOutOfBoundException, NullPointerException, ArithmeticException.
Example : Sample program for unchecked exception
class Uncheked_Demo
{
public static void main(String args[])
{
int a = 10;
int b = 0;
int c = a/b;
System.out.println(c);
}
}
Output:
Exception in thread "main"
java.lang.ArithmeticException: / by zero